How To Pull A Playlist From Youtube To React Native

YouTube gives content creators a ton of tools to add their piece of work for everyone to run across. But those tools only assist you lot manage the experience on YouTube.
How can we utilize the YouTube API to bring our video content to our ain website?
- YouTube has an API?
- What are nosotros going to build?
- Step 0: Getting started with Next.js
- Footstep one: Creating a Google Programmer project
- Step 2: Requesting playlist items from the YouTube API
- Stride 3: Showing YouTube playlist videos on the page
YouTube has an API?
Yup! YouTube, along with a lot of other Google services, has an API that we can use to have reward of our content in ways outside of YouTube. And the API is pretty all-encompassing.
With YouTube's API, you can practice things like manage your videos, access analytics, and manage playback. But nosotros're going to utilise it for grabbing videos from a playlist so that we can add them to a page.
What are nosotros going to build?
We're going to put together a Next.js app that uses the YouTube API To fetch videos from a playlist.

We'll take those videos and we'll brandish their thumbnails on a page that links to the video.
Pace 0: Getting started with Next.js
To spin up an app, we're going to utilise Adjacent.js. Using yarn or npm, we can easily create a new app that will let us dive correct into the code.
So to become started, navigate to where you want to create your projection and run:
yarn create next-app # or npx create-next-app
That script volition prompt you for a project name. I'm going to use "my-youtube-playlist", and it will install all of the dependencies needed to get started.
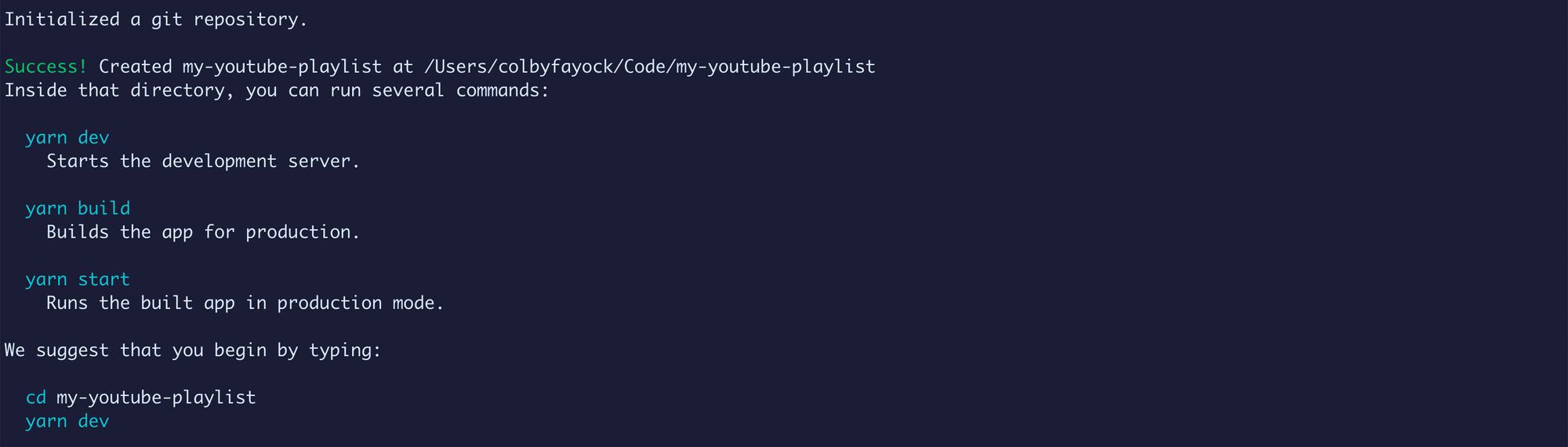
And then navigate to that directory and run yarn dev
to start your development server and we're set to go.
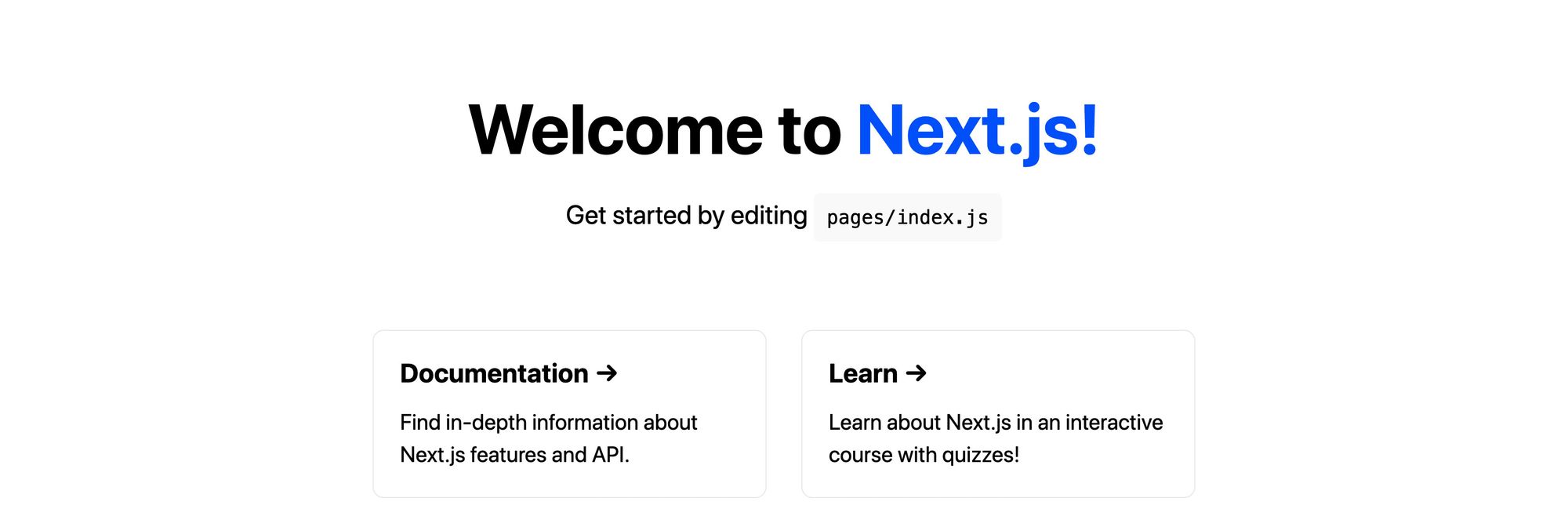
Follow along with the commit!
Pace 1: Creating a Google Programmer project
To use the API, nosotros're going to need a new project in the Google Developer console that volition allow the states to generate an API central to utilize the service.
First, head over to: https://console.developers.google.com/.
Once there and logged in with your Google account, we'll want to create a new project.
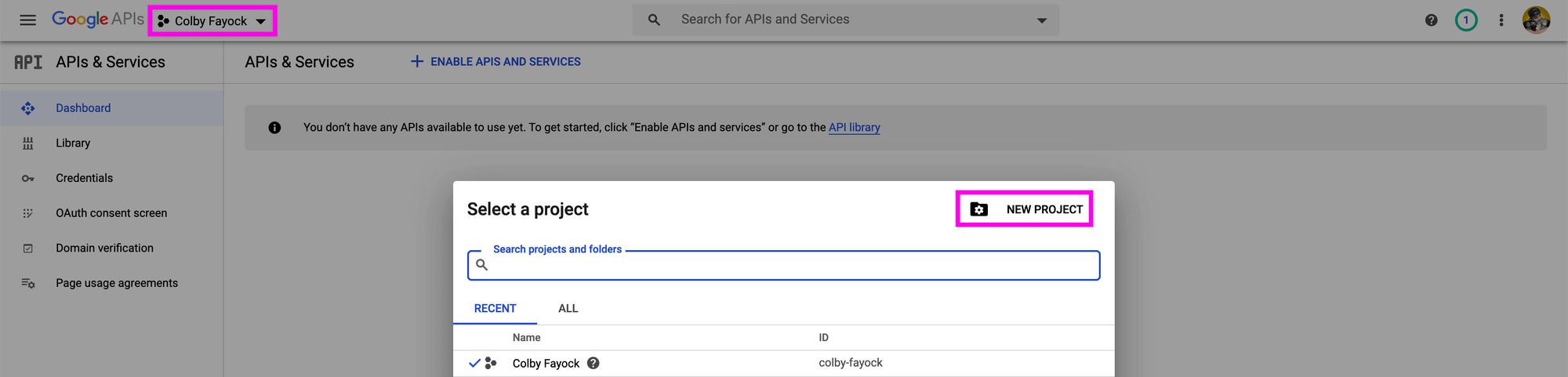
At this signal, you can proper name your projection any you'd like. I'm going with "My YouTube Playlist". Then click Create.
By default, Google won't drib you into the new projection. It kicks off a job to create that projection, but once it finishes, y'all can open back up the project selector and select your new project.

Next, we want to create an API central. Navigate to Credentials, click Create Credentials, so select API key.

This will create a new API key that you'll want to copy and save for after.

Note: This API key should be kept hush-hush. If y'all expose this or add it somewhere bachelor to the public like GitHub, others tin can abuse it and leave y'all with a neb to pay.
Finally, we need to add the YouTube API equally a service. Navigate to Library in the sidebar, search for "YouTube", then select YouTube Data API v3.

Finally subsequently that page loads, click Enable, which will bring you to a new dashboard page and you'll be gear up to get.

Stride 2: Requesting playlist items from the YouTube API
To request our data, we're going to use the getServerSideProps function.
Outset, open upward the pages/index.js
file and add together the following above the Dwelling house
component.
const YOUTUBE_PLAYLIST_ITEMS_API = 'https://www.googleapis.com/youtube/v3/playlistItems'; export async function getServerSideProps() { const res = await fetch(`${YOUTUBE_PLAYLIST_ITEMS_API}`) const data = await res.json(); return { props: { data } } }
Here's what we're doing:
- Nosotros're creating a new constant that stores our API endpoint
- We're creating and exporting a new
getServerSideProps
office - Inside that function, nosotros fetch our endpoint
- We and so transform that to JSON
- Finally, we return the information as props in our object
Now, if we destructure the information
prop from Dwelling house
and console log out that information like the following:
export default function Dwelling house({ data }) { console.log('data', information);
Nosotros tin can at present see that we become an error:

We're not using our API cardinal, so let's employ that.
Create a new file in the root of the project called .env.local
and add the following content:
YOUTUBE_API_KEY="[API Key]"
Make certain to supersede [API Key]
with your API key from Step 1.
At this point, you'll want to restart your server then Next.js can see the new variable.
Now, update the getServerSideProps
function to add your fundamental to the API request:
export async function getServerSideProps() { const res = await fetch(`${YOUTUBE_PLAYLIST_ITEMS_API}?key=${process.env.YOUTUBE_API_KEY}`)
And if we reload the page, again, we get an error:

Now, we're missing the playlist ID and the filters we want to grab our information.
Using the parameters from the playlist items API, let's update our API endpoint again:
const res = wait fetch(`${YOUTUBE_PLAYLIST_ITEMS_API}?part=snippet&maxResults=50&playlistId=[Playlist ID]&primal=${procedure.env.YOUTUBE_API_KEY}`)
Here, we're adding:
-
role=snippet
: this tells the API we want the snippet -
maxResults=50
: this sets the maximum number of playlist items that go returned to 50 -
playlistId=[Playlist ID]
: this adds the parameter to configure the playlist ID. Hither, you should update that value to the playlist ID of your choice.
Note: you tin can observe the playlist ID in the URL of the playlist yous want to apply.
And finally, when we reload the page, we have our data:
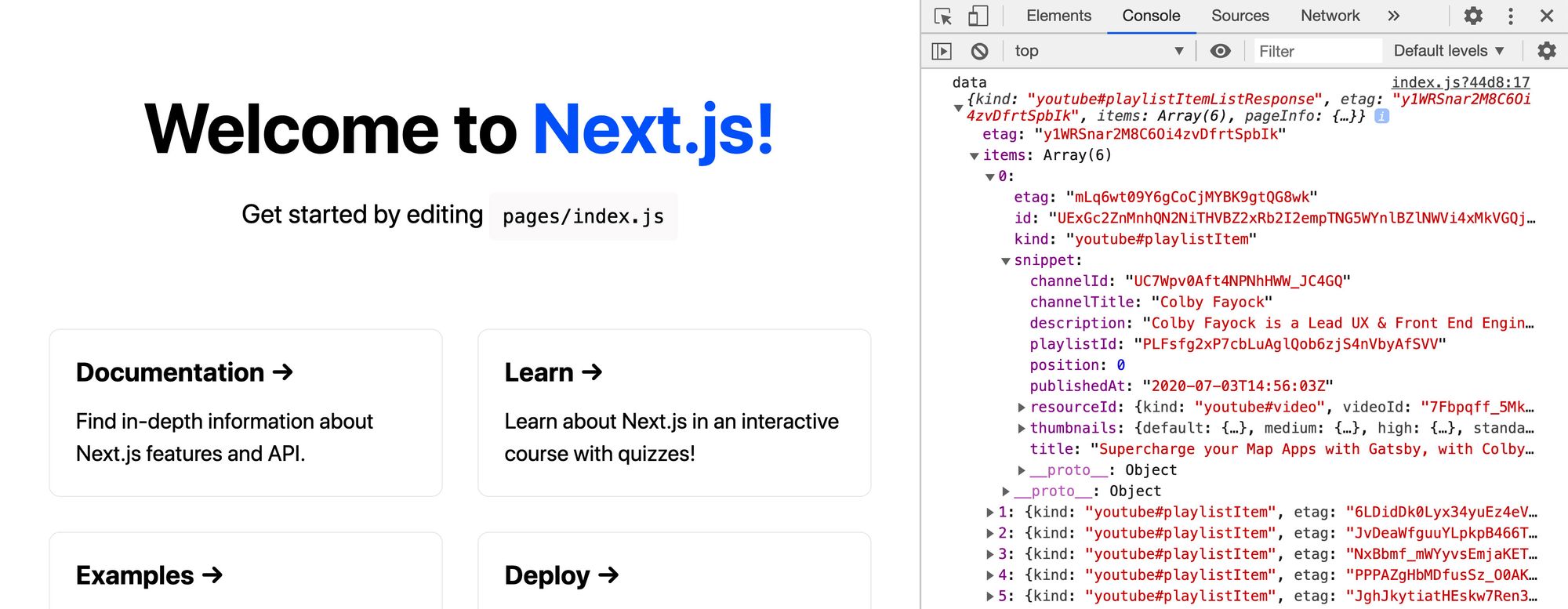
Follow along with the commit!
Pace 3: Showing YouTube playlist videos on the folio
Now that we accept our data, we want to display it.
To make use of what nosotros already accept from Next.js, we tin transform the existing grid into a grid of YouTube thumbnails.
Permit's supplant the entire <div>
with the className
of styles.grid
to:
<ul className={styles.grid}> {data.items.map(({ id, snippet = {} }) => { const { championship, thumbnails = {}, resourceId = {} } = snippet; const { medium } = thumbnails; return ( <li fundamental={id} className={styles.carte}> <a href={`https://www.youtube.com/watch?v=${resourceId.videoId}`}> <p> <img width={medium.width} height={medium.height} src={medium.url} alt="" /> </p> <h3>{ championship }</h3> </a> </li> ) })} </ul>
Here'south what we're doing:
- We're changing the
<div>
to a<ul>
then information technology'south more than semantic - We create a map through the items of our playlist to dynamically create new items
- We're destructuring our data grabbing the id, title, thumbnail, and video ID
- Using the ID, we add a key to our
<li>
- We also movement the
className
ofstyles.menu
from the<a>
to the<li>
- For our link, we apply the YouTube video ID to create the video URL
- We add our thumbnail image
- We apply our
title
for the<h3>
And if nosotros reload the folio, we can see that our videos are in that location, only the styles are a bit off.
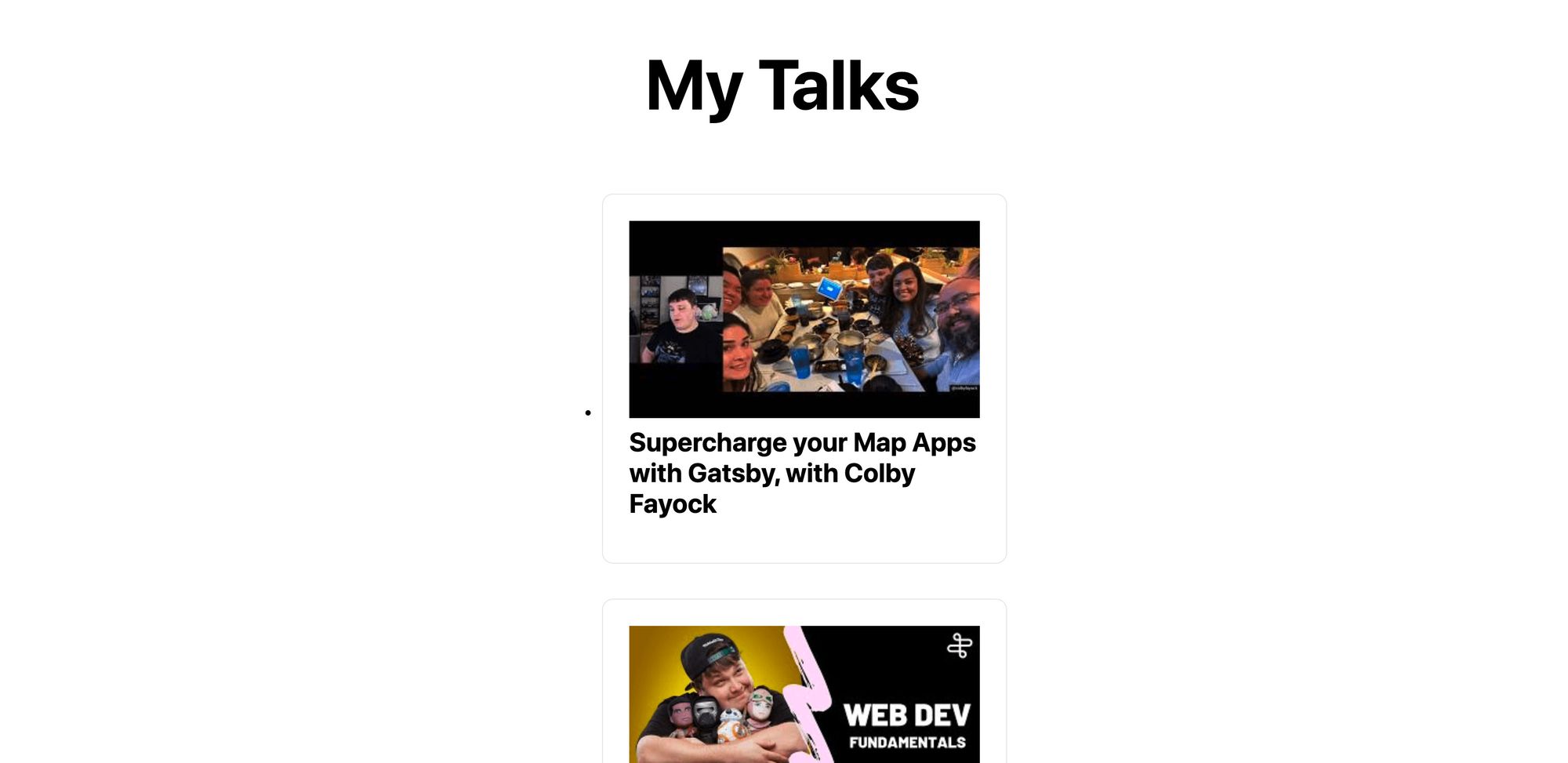
To fix this, we can start by adding the following styles to .grid
inside of the Home.module.css
file:
list-style: none; padding: 0; margin-left: 0;
We can too modify
marshal-items: center;
to
align-items: flex-start;
which will assistance gear up the alignment of our videos.
Only we'll notice we even so don't have two columns like the original Adjacent.js layout.

Under our .grid
course that nosotros just updated, add the following:
.grid img { max-width: 100%; }
This makes sure all of our images don't exceed the container. This volition prevent the overflow and volition let our images settle in to two columns.

And that gives us our playlist videos.
Follow along with the commit!
What else can we do?
Embed a role player and testify that video on click
YouTube as well gives controls for their own player. So using some JavaScript, we tin can take reward and when someone clicks ane of our video thumbnails, we can show an embedded player and play that video.
YouTube Player API Reference for iframe Embeds
Get analytics data
While YouTube provides some analytics in its dashboard, maybe you want something more than advanced.
You tin can use the Analytics and Reporting APIs to become more insight into your channel and videos.
YouTube Analytics and Reporting APIs
- ? Follow Me On Twitter
- ?️ Subscribe To My Youtube
- ✉️ Sign Upwardly For My Newsletter
Acquire to code for free. freeCodeCamp's open up source curriculum has helped more than 40,000 people get jobs as developers. Get started
Source: https://www.freecodecamp.org/news/how-to-add-a-youtube-playlist-to-a-nextjs-react-app-with-the-youtube-api/
Posted by: troupeingthe.blogspot.com
0 Response to "How To Pull A Playlist From Youtube To React Native"
Post a Comment